Understanding JavaScript Hoisting
Hoisting is a concept that describes how JavaScript stores variable and function declarations in memory during the compilation phase. For variables declared with var
, hoisting moves these declarations to the top of their function scope during compilation. However, while variable declarations are hoisted, their values are not assigned. This means if you access such a variable before its assignment, you’ll get undefined
.
When using variables declared with let/const
before their declaration, we encounter a ReferenceError
. This might lead people to believe that let/const
don’t hoist. In fact, let/const
do exhibit hoisting behavior, but with two key differences from var
:
-
Variables declared with
var
are hoisted to function scope, whereaslet/const
variables are only hoisted to block scope. -
During hoisting,
var
automatically initializes variables with the valueundefined
. In contrast,let/const
declarations are hoisted without initialization. This “uninitialized” state is commonly referred to as the Temporal Dead Zone (TDZ). The TDZ error was primarily designed forconst
variables. Ifconst
followed the same hoisting behavior asvar
, using aconst
variable before its declaration would returnundefined
. However, since constants should never change value within their scope, initially returningundefined
and later a different value would violate this principle. The TDZ error prevents this inconsistency.
Function declarations are also hoisted. Unlike variables, function hoisting creates the complete function object, allowing you to call functions before they appear in your code.
It’s important to note that function expressions behave differently. Their hoisting behavior matches the variable type they’re declared with. For example, if a function foo
is declared as a variable with var
, accessing it before the declaration will yield undefined
, and attempting to call undefined
results in an error:
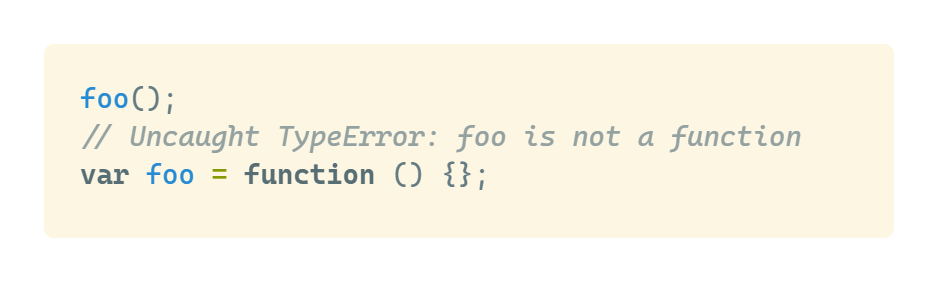
Similarly, for a function foo
declared with let
, accessing it before declaration places it in the temporal dead zone, resulting in a reference error:
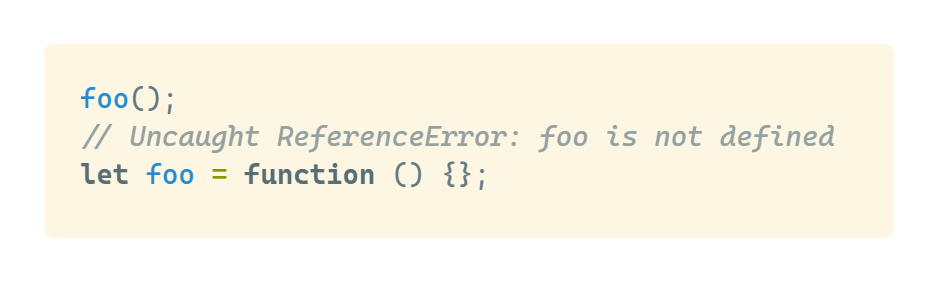