Understanding splice, slice, and split in JavaScript
When looking at splice, slice, and split individually, they might not seem confusing. However, once you’ve encountered all three, they start to feel familiar yet distinct, making it difficult to differentiate between them. They’re like the “Dango Three Brothers” - without careful examination, it’s easy to confuse which one to use in different situations.
The “Dango Three Brothers” is a song from the Japanese NHK children’s educational program “Fun with Mom” released in January 1999. It became extremely popular in Japan. The song style is super cute and pleasant to listen to - I recommend giving it a try to relieve some stress!
Table of Contents
- First Brother: splice
- Second Brother: slice
- Third Brother: split
- Why can slice operate on both arrays and strings, while splice can only operate on arrays but not strings?
First Brother: splice
mdn explanation (Array.prototype.splice()):
The splice() method changes the contents of an array by removing or replacing existing elements and/or adding new elements in place.
Before explaining splice’s functionality, I want to highlight something important to remember when using array methods: it’s crucial to know what type they return and how they operate. For example, does it return an “array” or a “string”? Does it return “a new array” or “modify the original array”? Knowing this helps prevent writing incorrect code.
Let’s get started!
splice can perform two operations on the original array: 1. Insert elements, and 2. Delete elements—or do both simultaneously → It modifies the original array and you can define a new variable name to capture the “deleted elements”. If no elements are deleted, it returns an empty array.
Let’s look at some examples:
Starting from index 2, delete 0 elements and insert “a”:
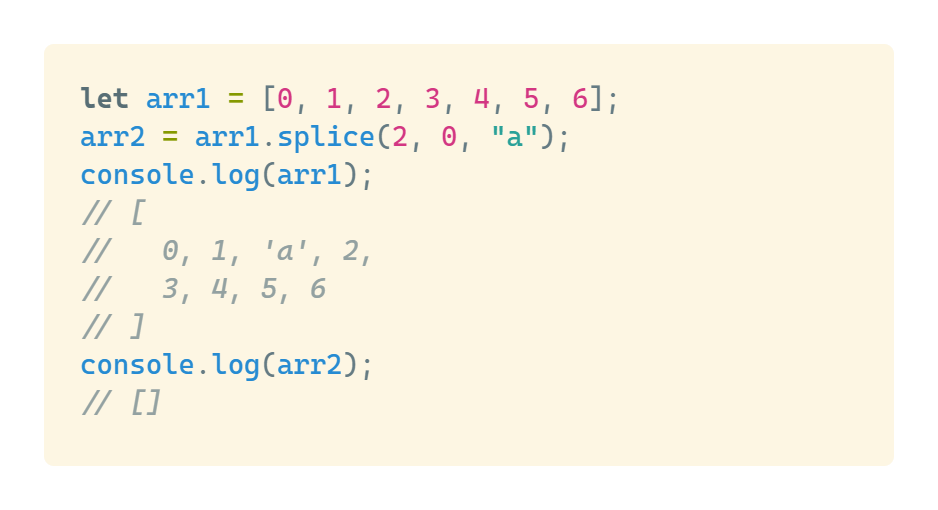
Starting from index 2, delete 1 element:
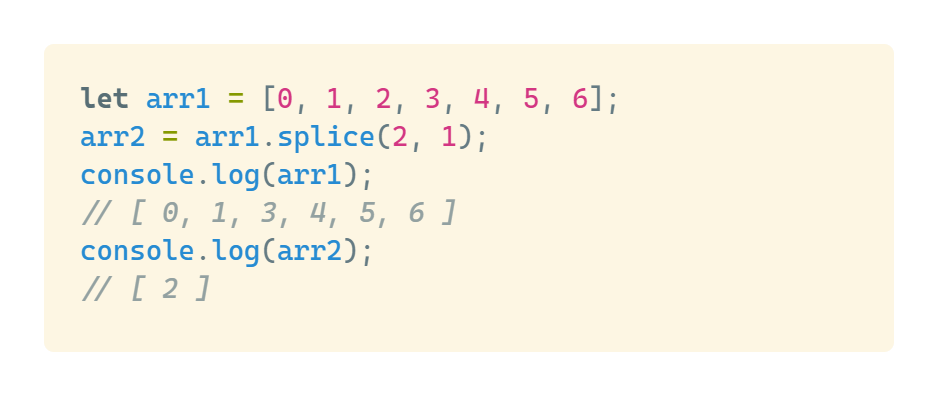
Starting from index 2, delete 1 element and insert “a”:
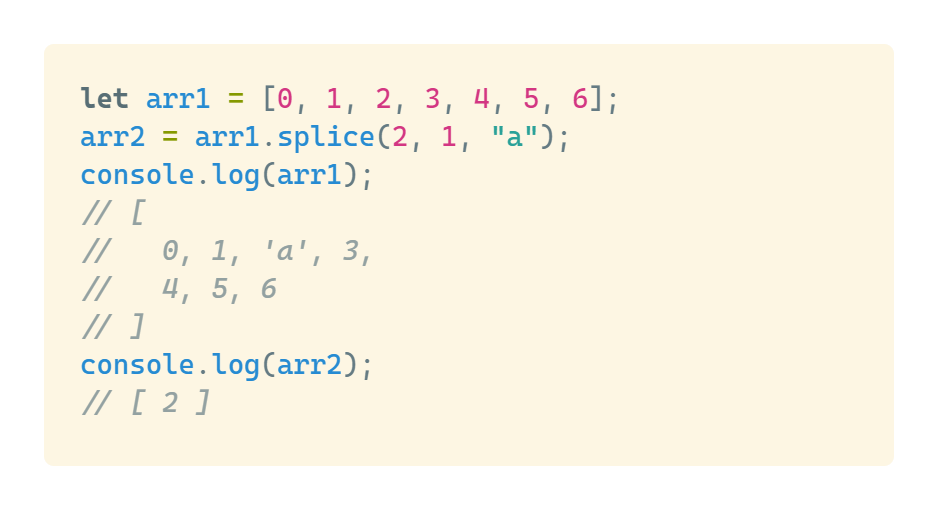
Starting from index 2, delete 1 element and insert “a, b, c”:
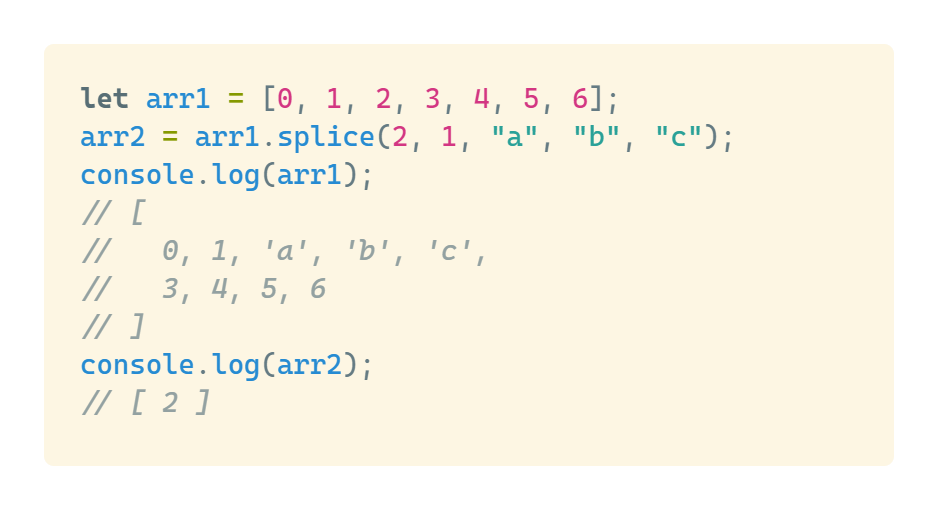
Second Brother: slice
mdn explanation (Array.prototype.slice()):
The slice() method returns a shallow copy of a portion of an array into a new array object selected from start to end (end not included) where start and end represent the index of items in that array. The original array will not be modified.
mdn explanation (String.prototype.slice()):
The slice() method extracts a section of a string and returns it as a new string, without modifying the original string.
slice can extract a portion of an “array” or “string” → It returns a new “array” or “string”, which is the extracted segment, while the original “array” or “string” remains unchanged.
Let’s look at some examples:
Slice from position 3 onwards:
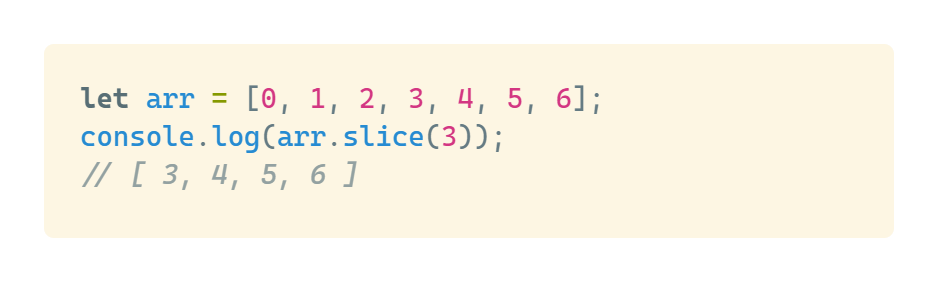
Slice from position 3 to position 6 (not including position 6):
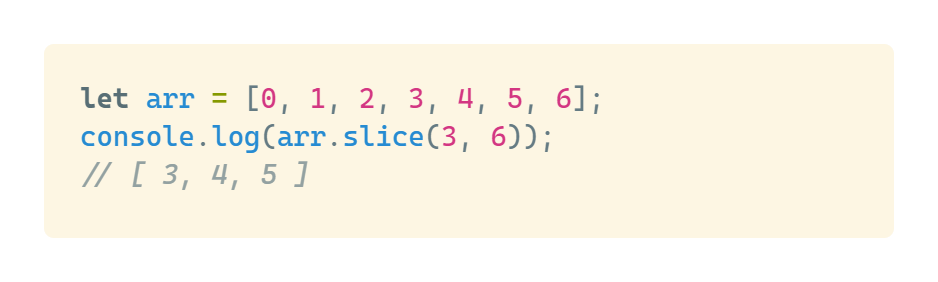
I’ve only provided examples of extracting from “arrays” above. For extracting from “strings”, please use your imagination and apply the same principles. (Translation: I’m too lazy to write more examples 😂)
Third Brother: split
mdn explanation (String.prototype.split()):
The split() method takes a pattern and divides a String into an ordered list of substrings by searching for the pattern, puts these substrings into an array, and returns the array.
split’s function is to “divide a string into an array”. When using it, you specify which character(s) in the original string to use as a separator → It returns a new “array”, while the original “string” remains unchanged.
Let me explain with the following example:
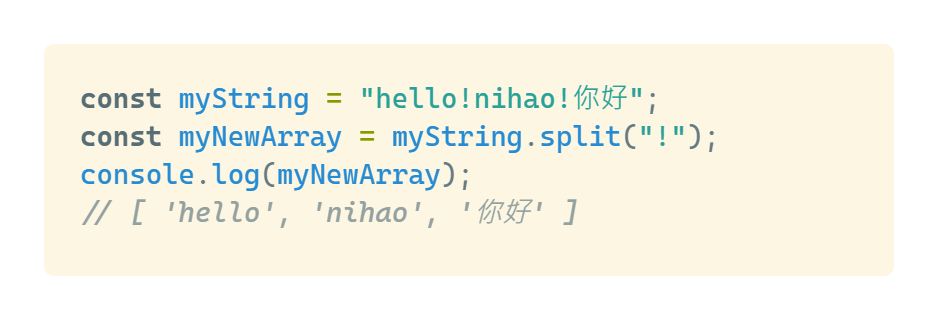
Note that the separator used will disappear in the returned new “array”. In the example above, we specified ”!” as the separator, so the exclamation marks don’t appear in the resulting array elements.
This method is particularly useful when processing .csv files! (.csv is one of the most common export formats for Excel or Google Sheets, which converts spreadsheet fields into a text file with comma-separated values)
Let’s also look at the demo provided by mdn:
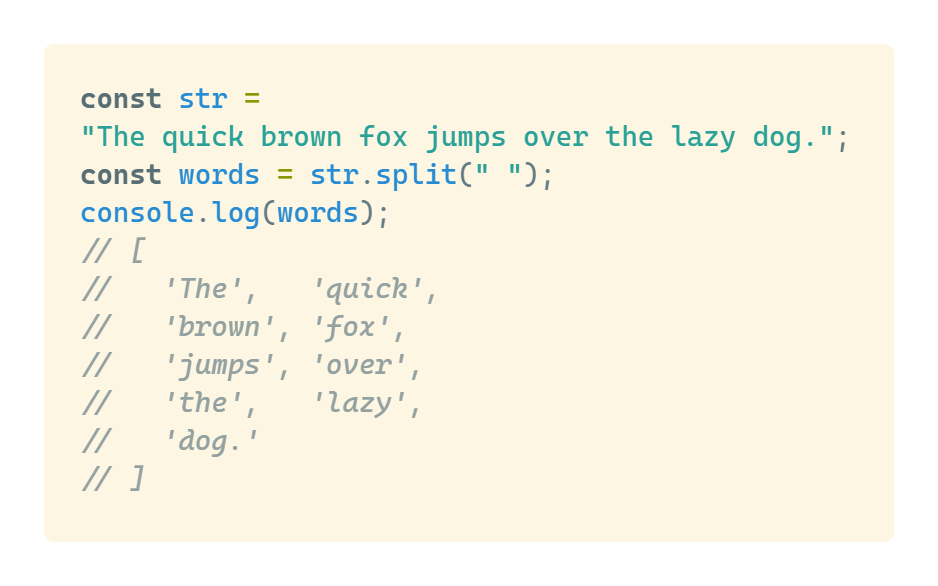
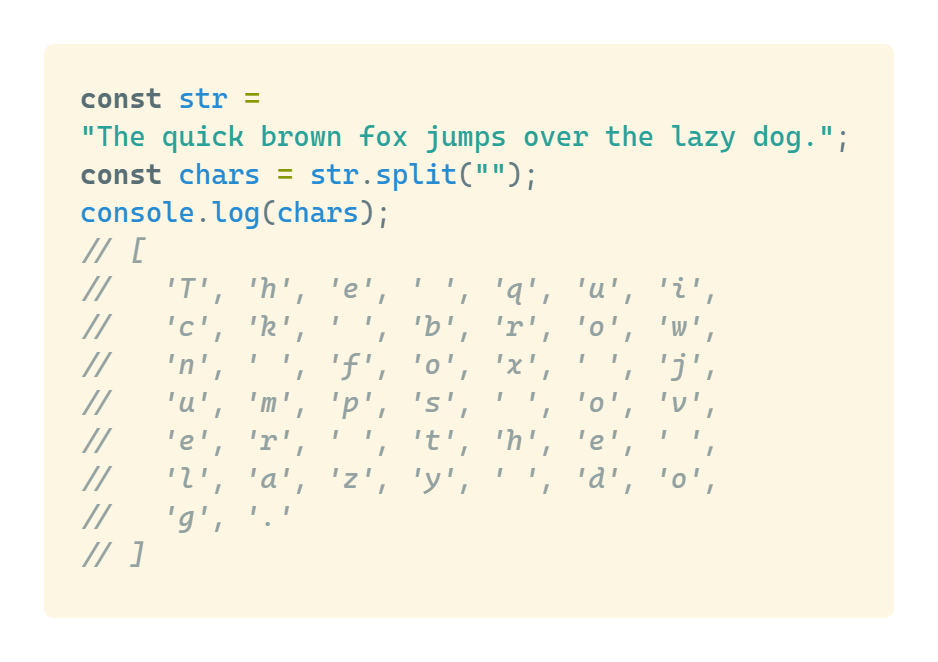
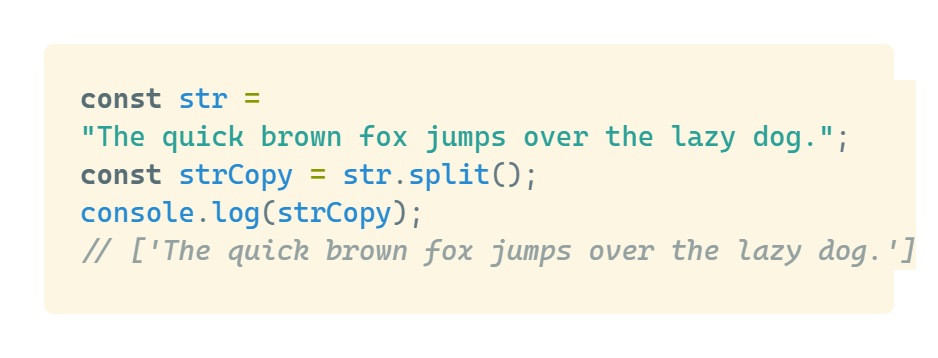
Here we can see that if the original string is a complete sentence, using ”’ ’” (with a space in between) as the separator will split it into individual words, using ””” (with no space in between) as the separator will split it into individual letters, and using "" (not putting anything) as the separator will put the entire original string (in this case, a complete sentence) directly into the new array as its only element.
Well, our “spot the difference” game ends here for now. There are probably many more features I haven’t listed, but I’ll leave those for another time if I happen to encounter them.
Why can slice operate on both arrays and strings, while splice can only operate on arrays but not strings?
This was a question that came to mind after playing the “spot the difference” game. My current understanding is that functions that can operate on arrays should typically also be able to operate on strings, as this would be a win-win situation. But why can splice only operate on arrays?
This is because in JavaScript, primitive types (including strings) are immutable, meaning you cannot directly modify the content of primitive type variables. Note that this refers to not being able to directly call any built-in functions to make modifications, but you can still reassign values to change the content. Here’s a simple example to illustrate:
If a variable is of primitive type, calling any built-in function alone won’t change the variable’s value, e.g.,
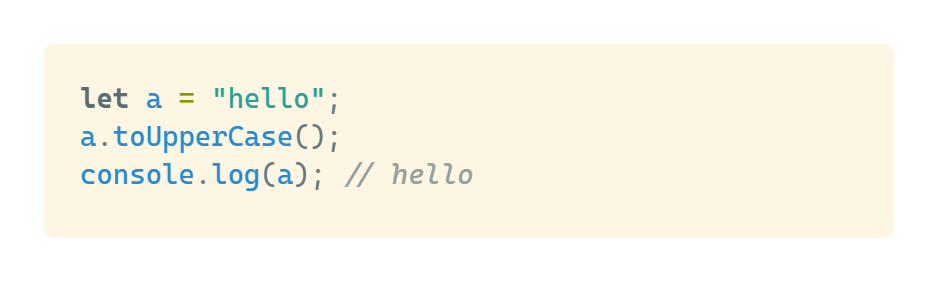
You must reassign the value for a to change:
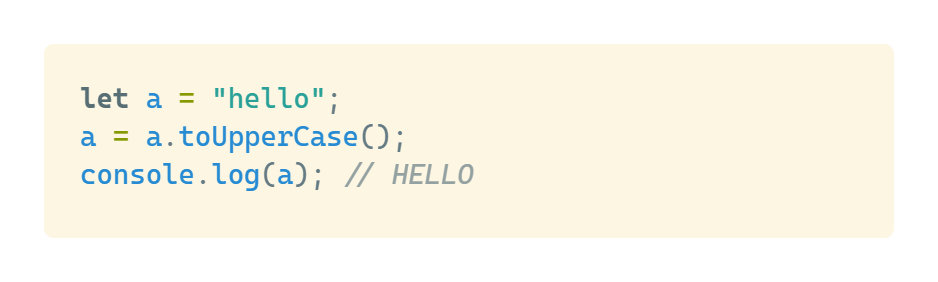
Back to the original question, we know that the splice function itself is designed to directly change the original value, which would contradict JavaScript’s immutability principle for primitive types. This is why the splice function cannot operate on strings. Arrays in JavaScript are objects, which don’t have the immutability restriction, so directly manipulating the original value is possible. Here’s a simple example to illustrate:
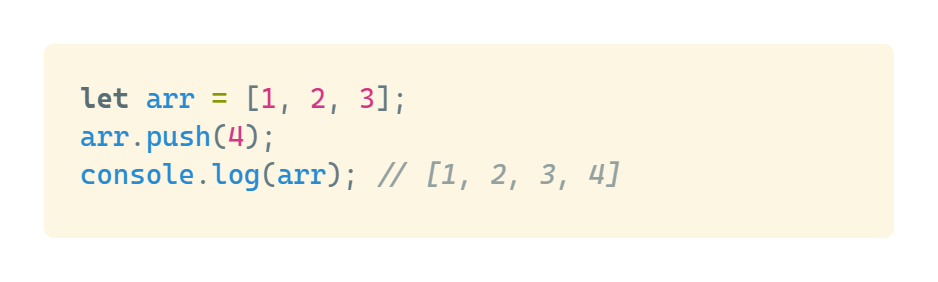
From the example above, we can see that if a variable is of Object type, directly calling built-in functions will change the variable’s value.
As for the slice function, since its functionality doesn’t change the original value, there’s no contradiction as mentioned above, so it works fine for both array and string operations.